PHP Arrays Explained: Types, Syntax, and Practical Examples
Arrays are a fundamental data structure in PHP, allowing you to store and manipulate collections of data. In this article, we’ll delve into the world of PHP arrays, exploring their types, syntax, and practical examples.
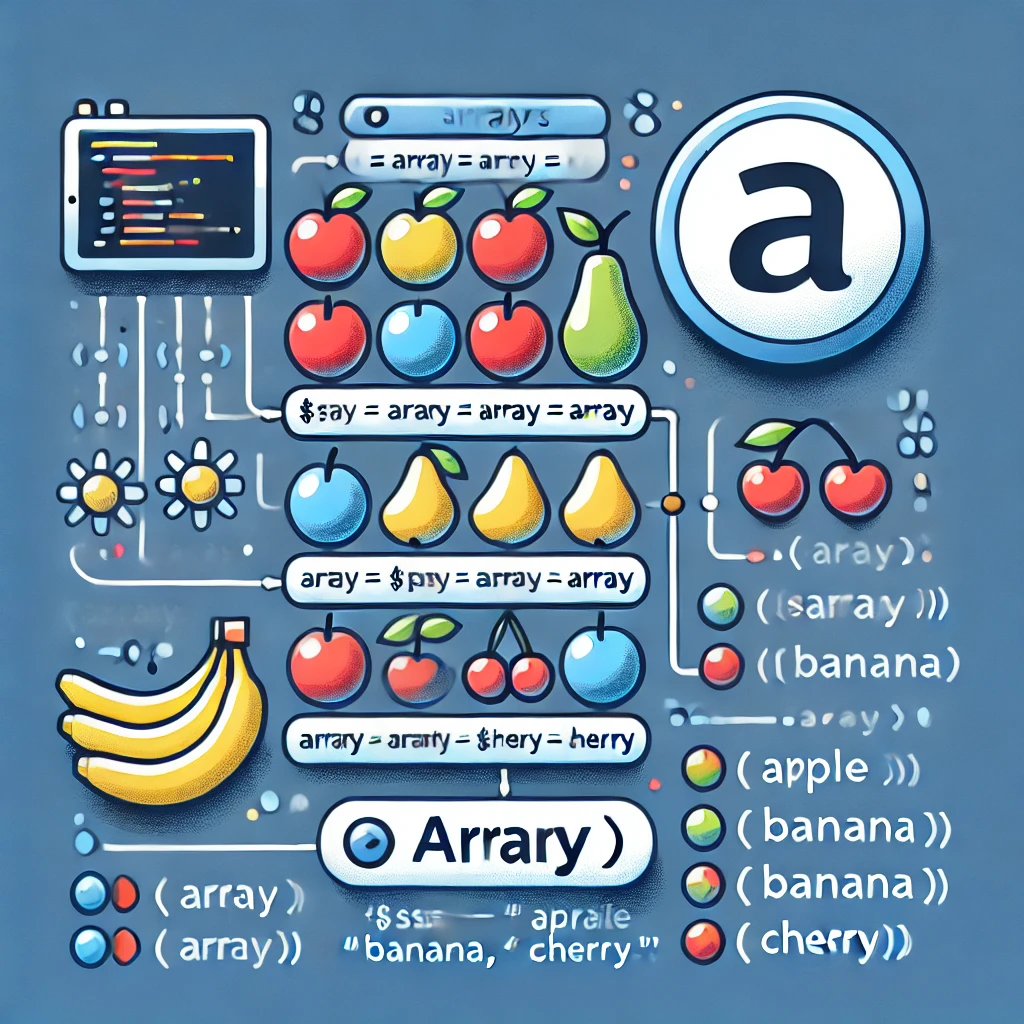
What are PHP Arrays?
In PHP, an array is a data structure that stores a collection of elements, each identified by a unique key or index. Arrays can contain a mix of data types, including strings, integers, floats, and other arrays.
Types of PHP Arrays
PHP supports two types of arrays:
1. Indexed Arrays
Indexed arrays are the most common type of array in PHP. They use a numerical index to store and retrieve elements.
2. Associative Arrays
Associative arrays, also known as hash tables, use a string key to store and retrieve elements.
3. Multidimensional Arrays
Multidimensional arrays are arrays that contain other arrays as elements.
PHP Array Syntax
The syntax for creating an array in PHP is as follows:PHP
$array_name = array(
'key' => 'value',
'key2' => 'value2',
// ...
);
Alternatively, you can use the square bracket syntax (available in PHP 5.4 and later):PHP
$array_name = [
'key' => 'value',
'key2' => 'value2',
// ...
];
Creating Indexed Arrays
To create an indexed array, simply omit the key and assign the value:PHP
$fruits = array('apple', 'banana', 'orange');
Or, using the square bracket syntax:PHP
$fruits = ['apple', 'banana', 'orange'];
Creating Associative Arrays
To create an associative array, specify the key and value:PHP
$person = array(
'name' => 'John Doe',
'age' => 30,
'city' => 'New York'
);
Or, using the square bracket syntax:PHP
$person = [
'name' => 'John Doe',
'age' => 30,
'city' => 'New York'
];
Accessing Array Elements
To access an array element, use the following syntax:PHP
$array_name['key'];
For example:PHP
echo $fruits[0]; // Output: apple
echo $person['name']; // Output: John Doe
Manipulating Arrays
PHP provides several functions for manipulating arrays, including:
array_push()
: Adds one or more elements to the end of an array.array_pop()
: Removes the last element from an array.array_shift()
: Removes the first element from an array.array_unshift()
: Adds one or more elements to the beginning of an array.array_merge()
: Merges two or more arrays into a single array.array_slice()
: Extracts a portion of an array.
Practical Examples
Example 1: Shopping Cart
Suppose you’re building an e-commerce website and you want to store a user’s shopping cart items in an array:PHP
$cart = array(
'product1' => array('name' => 'Product 1', 'price' => 10.99),
'product2' => array('name' => 'Product 2', 'price' => 5.99),
'product3' => array('name' => 'Product 3', 'price' => 7.99)
);
You can then access and manipulate the cart items using array functions:PHP
echo $cart['product1']['name']; // Output: Product 1
array_push($cart, array('product4' => array('name' => 'Product 4', 'price' => 12.99)));
Example 2: Student Grades
Suppose you’re building a student grading system and you want to store a student’s grades in an array:PHP
$grades = array(
'math' => 85,
'science' => 90,
'english' => 78
);
You can then access and manipulate the grades using array functions:PHP
echo $grades['math']; // Output: 85
array_push($grades, array('history' => 92));
Conclusion
In this article, we’ve explored the world of PHP arrays, covering their types, syntax, and practical examples. Arrays are a fundamental data structure in PHP, and understanding how to work with them is essential for building robust and efficient web applications. Whether you’re building a shopping cart, a student grading system, or any other type of web application

Kushagra Kumar Mishra

Latest posts by Kushagra Kumar Mishra (see all)
- Advanced WordPress Topics: Security, Performance, and Scalability (Part-2) - March 10, 2025
- Troubleshooting and Advanced Topics Part-1 - March 10, 2025
- Website Design and Development - March 10, 2025
Recent Comments