Tables in PHP: A Comprehensive Guide
Tables are a fundamental aspect of web development, especially when dealing with structured data. PHP, combined with MySQL and HTML, provides an excellent framework for creating, managing, and displaying data in tables. In this blog, we’ll cover:
- How to use
GET
andPOST
methods in PHP. - Creating and managing tables in MySQL.
- Displaying data in HTML tables using PHP.
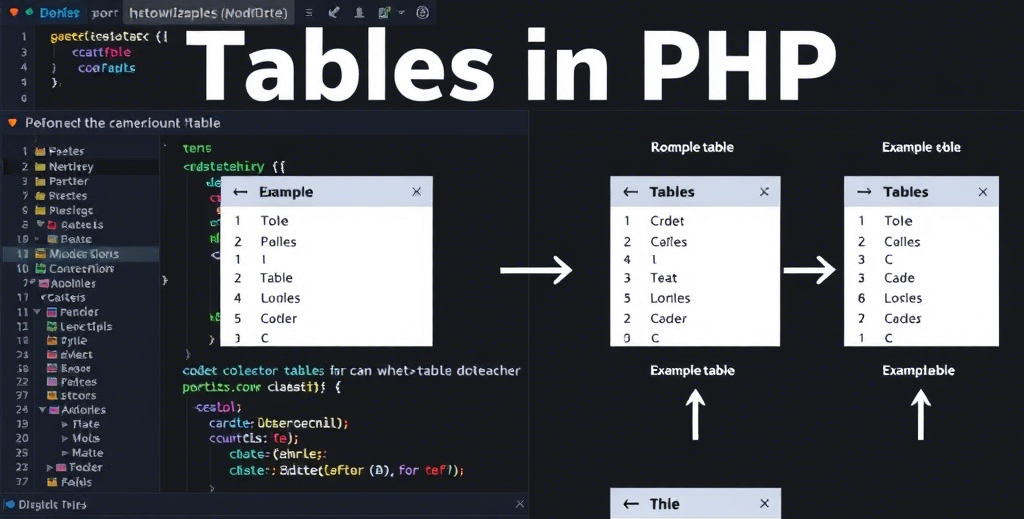
Understanding GET
and POST
Methods
GET
and POST
are the two most common HTTP methods used for transferring data between a client and a server.
GET
Method:
- Sends data through the URL.
- Ideal for requests where data is not sensitive (e.g., search queries).
- Limited by the URL length.
Example:
<?php
if (isset($_GET['name'])) {
$name = $_GET['name'];
echo "Hello, " . htmlspecialchars($name);
}
?>
<!-- HTML -->
<form method="GET">
<label for="name">Enter your name:</label>
<input type="text" id="name" name="name">
<button type="submit">Submit</button>
</form>
POST
Method:
- Sends data through the HTTP request body.
- More secure for sensitive data (e.g., passwords).
- No URL length limitations.
Example:
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST' && isset($_POST['email'])) {
$email = $_POST['email'];
echo "Your email is: " . htmlspecialchars($email);
}
?>
<!-- HTML -->
<form method="POST">
<label for="email">Enter your email:</label>
<input type="email" id="email" name="email">
<button type="submit">Submit</button>
</form>
Creating and Managing Tables in MySQL
MySQL tables store data in a structured format. Each table consists of rows and columns.
Creating a Table:
SQL Query:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100),
age INT
);
Inserting Data:
SQL Query:
INSERT INTO users (name, email, age) VALUES
('Alice', '[email protected]', 25),
('Bob', '[email protected]', 30),
('Charlie', '[email protected]', 35);
Fetching Data:
SQL Query:
SELECT * FROM users;
Displaying Data in HTML Tables Using PHP
To display data stored in a MySQL table, use PHP to fetch the data and dynamically generate an HTML table.
Example:
PHP Code:
<?php
include 'db.php';
$query = "SELECT * FROM users";
$result = $conn->query($query);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Users Table</title>
</head>
<body>
<h1>Users List</h1>
<table border="1">
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
<th>Age</th>
</tr>
<?php while ($row = $result->fetch_assoc()) : ?>
<tr>
<td><?php echo $row['id']; ?></td>
<td><?php echo $row['name']; ?></td>
<td><?php echo $row['email']; ?></td>
<td><?php echo $row['age']; ?></td>
</tr>
<?php endwhile; ?>
</table>
</body>
</html>
Explanation:
- Database Connection: Connect to the MySQL database using a
db.php
file. - Query Execution: Use
SELECT
to fetch data from theusers
table. - Dynamic Table Creation: Use PHP loops to populate table rows with data.
Combining GET
and POST
with Tables
You can enhance user interaction by integrating GET
and POST
methods with tables. For example, creating a form to filter data:
Filter Example:
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST' && isset($_POST['age_filter'])) {
$age_filter = (int) $_POST['age_filter'];
$query = "SELECT * FROM users WHERE age >= $age_filter";
} else {
$query = "SELECT * FROM users";
}
$result = $conn->query($query);
?>
<form method="POST">
<label for="age_filter">Filter by Age (>=):</label>
<input type="number" id="age_filter" name="age_filter">
<button type="submit">Filter</button>
</form>
<table border="1">
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
<th>Age</th>
</tr>
<?php while ($row = $result->fetch_assoc()) : ?>
<tr>
<td><?php echo $row['id']; ?></td>
<td><?php echo $row['name']; ?></td>
<td><?php echo $row['email']; ?></td>
<td><?php echo $row['age']; ?></td>
</tr>
<?php endwhile; ?>
</table>
Conclusion
Tables are an essential part of web development for managing and displaying data. By understanding the GET
and POST
methods, creating tables in MySQL, and dynamically displaying them in HTML using PHP, you can build powerful, data-driven web applications.

Kushagra Kumar Mishra

Latest posts by Kushagra Kumar Mishra (see all)
- Advanced WordPress Topics: Security, Performance, and Scalability (Part-2) - March 10, 2025
- Troubleshooting and Advanced Topics Part-1 - March 10, 2025
- Website Design and Development - March 10, 2025
Recent Comments