Understanding Encapsulation in PHP: Protect Your Data
Encapsulation is a key principle of Object-Oriented Programming (OOP) that ensures data safety and prevents unintended interference. By bundling the data (variables) and the methods (functions) that operate on the data into a single unit (class), encapsulation allows you to control access and modify behavior as needed.
In this blog, we will explore encapsulation in PHP, its benefits, and practical implementation.
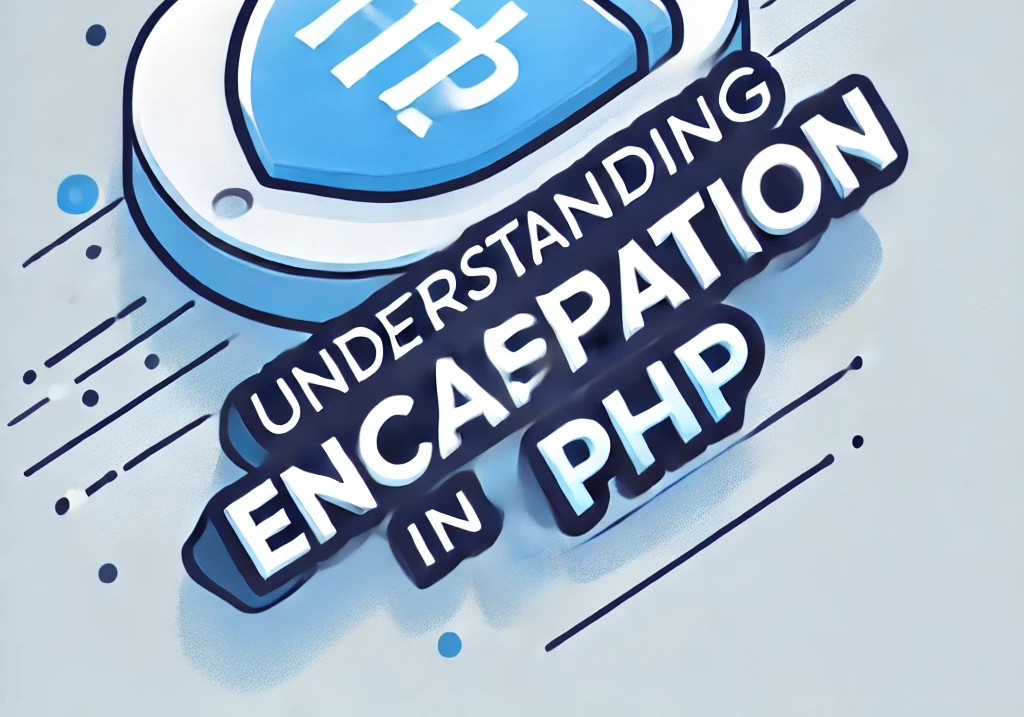
What is Encapsulation?
Encapsulation is the technique of restricting direct access to an object’s data and exposing only the necessary parts through well-defined methods. It enables better control over how data is accessed and modified, ensuring the integrity of the object.
In PHP, encapsulation is achieved using access modifiers:
- public: The property or method is accessible from anywhere.
- protected: The property or method is accessible only within the class itself and its subclasses.
- private: The property or method is accessible only within the class where it is defined.
Example of Encapsulation in PHP
Let’s see encapsulation in action with a real-world example of a banking system:
Code Example:
<?php
class BankAccount {
private $accountNumber;
private $balance;
public function __construct($accountNumber, $initialBalance) {
$this->accountNumber = $accountNumber;
$this->balance = $initialBalance;
}
// Getter method for balance
public function getBalance() {
return $this->balance;
}
// Method to deposit money
public function deposit($amount) {
if ($amount > 0) {
$this->balance += $amount;
echo "Deposited: $amount" . PHP_EOL;
} else {
echo "Invalid deposit amount." . PHP_EOL;
}
}
// Method to withdraw money
public function withdraw($amount) {
if ($amount > 0 && $amount <= $this->balance) {
$this->balance -= $amount;
echo "Withdrew: $amount" . PHP_EOL;
} else {
echo "Invalid withdrawal amount." . PHP_EOL;
}
}
}
$account = new BankAccount("12345678", 1000);
$account->deposit(500);
$account->withdraw(200);
echo "Balance: " . $account->getBalance() . PHP_EOL;
?>
Output:
Deposited: 500
Withdrew: 200
Balance: 1300
In this example:
- The $balance and $accountNumber properties are private, ensuring they cannot be accessed or modified directly from outside the class.
- Public methods like
deposit
,withdraw
, andgetBalance
provide controlled access to the properties.
Benefits of Encapsulation
- Data Protection: Prevents unauthorized access and accidental modification of critical data.
- Improved Maintenance: Encapsulated code is easier to debug, maintain, and extend.
- Controlled Access: You can define custom logic for how data is accessed or modified.
- Modularity: Bundling data and methods into a single class improves code organization and reusability.
Best Practices for Encapsulation in PHP
- Use Private and Protected Access Modifiers: Start with the least accessible modifier and increase visibility only when necessary.
- Implement Getter and Setter Methods: Use these methods to validate data before accessing or modifying private properties.
- Avoid Public Properties: Keep properties private or protected to maintain control over their behavior.
- Combine with Other OOP Principles: Leverage encapsulation alongside inheritance, polymorphism, and abstraction for robust design.
Encapsulation in PHP with Real-Life Analogy
Imagine a vending machine:
- The machine’s internal mechanisms (like counting coins, dispensing products, etc.) are hidden from the user.
- The user interacts only with the interface: inserting coins and selecting a product.
- The machine encapsulates its complexity while providing a simple interface for interaction.
Similarly, encapsulation in PHP hides the internal complexities of a class while providing a clean and user-friendly interface for interaction.
Conclusion
Encapsulation is a powerful concept in PHP that promotes data security, modularity, and maintainability. By restricting access to sensitive data and exposing only necessary functionalities, you can ensure the integrity and reliability of your applications.

Kushagra Kumar Mishra

Latest posts by Kushagra Kumar Mishra (see all)
- Advanced WordPress Topics: Security, Performance, and Scalability (Part-2) - March 10, 2025
- Troubleshooting and Advanced Topics Part-1 - March 10, 2025
- Website Design and Development - March 10, 2025
Recent Comments