Bootstrap in HTML: A Beginner’s Guide
Bootstrap is a powerful front-end framework that simplifies web development by providing ready-to-use CSS and JavaScript components. It helps create responsive and mobile-first websites quickly and efficiently.
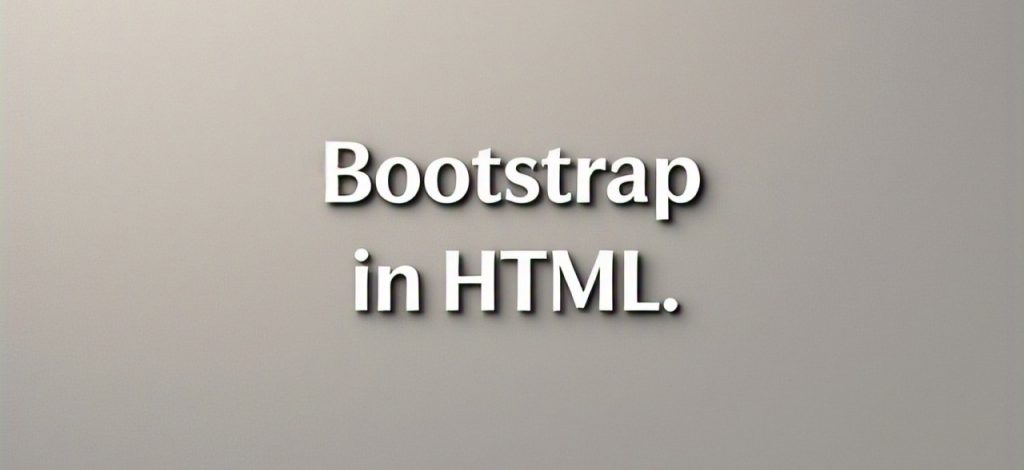
1. What is Bootstrap?
Bootstrap is an open-source framework developed by Twitter to make web development easier. It includes:
- Pre-designed CSS components
- JavaScript plugins
- A flexible grid system for responsive layouts
- Built-in utilities for styling
2. How to Include Bootstrap in Your HTML Project
There are two main ways to include Bootstrap in your project:
Using CDN (Recommended for Quick Setup)
Add the following lines in your HTML file’s <head>
section:
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- Bootstrap JavaScript -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
Downloading Bootstrap (Recommended for Customization)
- Download Bootstrap from getbootstrap.com.
- Extract and link Bootstrap files in your project:
<link rel="stylesheet" href="css/bootstrap.min.css">
<script src="js/bootstrap.bundle.min.js"></script>
3. Bootstrap Grid System
Bootstrap uses a 12-column grid system that makes it easy to create responsive layouts.
<div class="container">
<div class="row">
<div class="col-md-6">Column 1</div>
<div class="col-md-6">Column 2</div>
</div>
</div>
Key Grid Classes:
.container
– Defines a layout container..row
– Creates a row..col-md-6
– Creates a column that takes 6 out of 12 grid spaces on medium devices.
4. Bootstrap Components
1. Buttons
Bootstrap provides pre-styled buttons with various classes:
<button class="btn btn-primary">Primary Button</button>
<button class="btn btn-success">Success Button</button>
2. Navigation Bar
Create a responsive navbar easily:
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">My Website</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item"><a class="nav-link" href="#">Home</a></li>
<li class="nav-item"><a class="nav-link" href="#">About</a></li>
</ul>
</div>
</nav>
3. Forms
Bootstrap provides easy form styling:
<form>
<div class="mb-3">
<label class="form-label">Email address</label>
<input type="email" class="form-control">
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
5. Bootstrap Utilities
Bootstrap provides utility classes for styling without writing CSS:
- Spacing:
mt-3
(margin top),p-2
(padding) - Text:
text-center
,text-uppercase
- Colors:
bg-primary
,text-danger
6. Responsive Design with Bootstrap
Bootstrap makes it easy to create mobile-friendly layouts:
<div class="container">
<div class="row">
<div class="col-lg-4 col-md-6 col-sm-12">Responsive Column</div>
</div>
</div>
This layout adjusts based on screen size:
col-lg-4
(4 columns on large screens)col-md-6
(6 columns on medium screens)col-sm-12
(full width on small screens)
7. Conclusion
Bootstrap simplifies web development with its grid system, pre-styled components, and utilities. Whether you’re a beginner or an experienced developer, Bootstrap helps create stunning and responsive websites efficiently.
In the next guide, we will explore JavaScript in HTML, covering the basics of adding interactivity to web pages!

Kushagra Kumar Mishra

Latest posts by Kushagra Kumar Mishra (see all)
- Advanced WordPress Topics: Security, Performance, and Scalability (Part-2) - March 10, 2025
- Troubleshooting and Advanced Topics Part-1 - March 10, 2025
- Website Design and Development - March 10, 2025
Recent Comments