Forms in HTML: A Complete Guide
Forms are essential in web development as they allow users to input and submit data. Whether it’s for login pages, contact forms, or search functionalities, mastering HTML forms is crucial for interactive web applications.
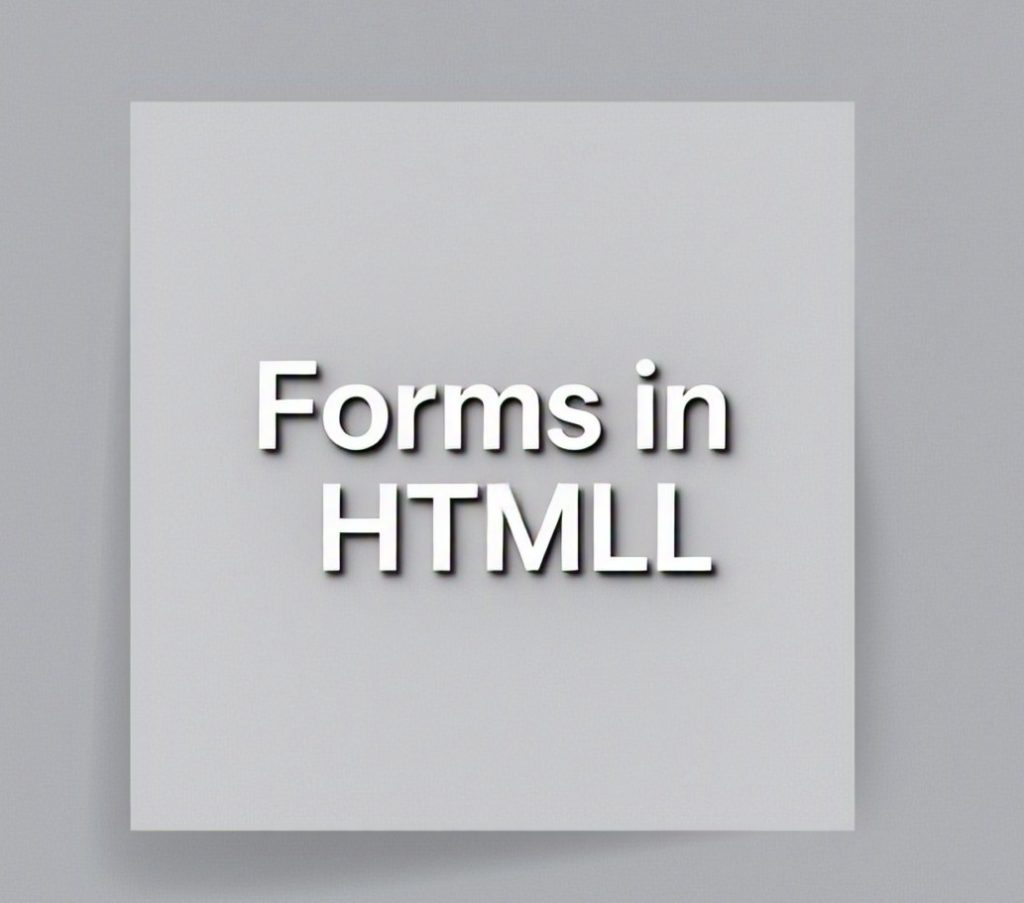
What is an HTML Form?
An HTML form collects user input and sends it to a server for processing. It is defined using the <form>
tag and contains form elements such as text fields, checkboxes, radio buttons, and buttons.
Basic Structure of an HTML Form
<form action="submit.php" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<input type="submit" value="Submit">
</form>
Attributes of the <form>
Tag:
action
– Specifies where to send the form data.method
– Defines the HTTP method (GET
orPOST
).target
– Determines how the response is displayed (_self
,_blank
).
Common HTML Form Elements
1. Input Fields
The <input>
tag is used to collect user data.
<input type="text" name="username" placeholder="Enter your name">
2. Radio Buttons & Checkboxes
Used for selecting options.
<input type="radio" name="gender" value="male"> Male
<input type="radio" name="gender" value="female"> Female
<input type="checkbox" name="subscribe" value="yes"> Subscribe to newsletter
3. Dropdown Menus
Allows users to select from multiple options.
<select name="country">
<option value="india">India</option>
<option value="usa">USA</option>
<option value="uk">UK</option>
</select>
4. Textareas
For multi-line text input.
<textarea name="message" rows="4" cols="50">Enter your message...</textarea>
5. Buttons
Used to submit or reset forms.
<button type="submit">Submit</button>
<button type="reset">Reset</button>
Form Validation
Validation ensures that users enter correct information.
1. Required Fields
<input type="text" name="username" required>
2. Email Validation
<input type="email" name="email" required>
3. Min and Max Length
<input type="text" name="username" minlength="3" maxlength="20">
4. Pattern Matching
<input type="text" name="zipcode" pattern="[0-9]{5}" title="Enter a 5-digit ZIP code">
Enhancing Forms with CSS
CSS improves form appearance.
input, textarea, select {
width: 100%;
padding: 10px;
margin: 5px 0;
border-radius: 5px;
}
button {
background-color: blue;
color: white;
padding: 10px;
border: none;
}
Conclusion
Forms are a critical component of web development, allowing interaction between users and servers. Understanding various form elements, attributes, and validation techniques is essential. In the next guide, we will explore Gallery Creation in HTML and CSS to build image galleries effectively.

Kushagra Kumar Mishra

Latest posts by Kushagra Kumar Mishra (see all)
- Advanced WordPress Topics: Security, Performance, and Scalability (Part-2) - March 10, 2025
- Troubleshooting and Advanced Topics Part-1 - March 10, 2025
- Website Design and Development - March 10, 2025
Recent Comments