Image Carousel in HTML
An image carousel is a slideshow that allows users to cycle through images automatically or manually. It enhances user experience by displaying multiple images in a compact space.
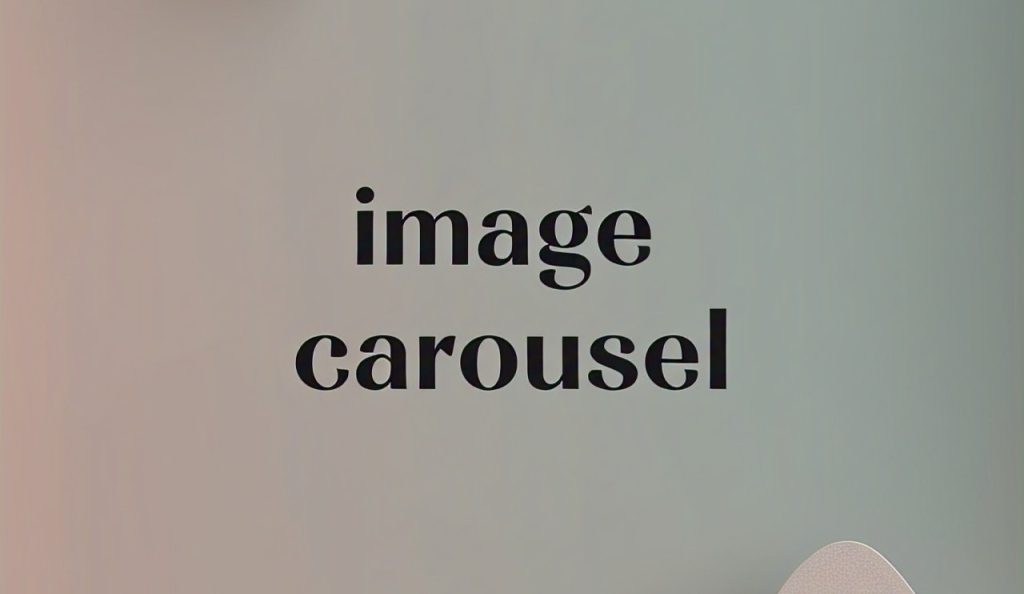
1. What is an Image Carousel?
An image carousel displays a series of images in a rotating manner. It is widely used in websites for showcasing portfolios, product galleries, and advertisements.
2. How to Create an Image Carousel in HTML
To create a simple image carousel, we can use HTML, CSS, and JavaScript. Alternatively, Bootstrap provides a built-in carousel component.
Basic HTML Structure
< div class = "carousel" > < img src = "image1.jpg" class = "carousel-image" alt = "Image 1" > < img src = "image2.jpg" class = "carousel-image" alt = "Image 2" > < img src = "image3.jpg" class = "carousel-image" alt = "Image 3" > < button class = "prev" onclick = "prevSlide()" >❮</ button > < button class = "next" onclick = "nextSlide()" >❯</ button > </ div > |
CSS for Styling
.carousel { position : relative ; max-width : 600px ; overflow : hidden ; } .carousel-image { width : 100% ; display : none ; } .carousel-image:first-child { display : block ; } button { position : absolute ; top : 50% ; transform : translateY ( -50% ); background : rgba ( 0 , 0 , 0 , 0.5 ); color : white ; border : none ; padding : 10px ; } .prev { left : 0 ; } .next { right : 0 ; } |
JavaScript for Functionality
let currentIndex = 0; const images = document.querySelectorAll( ".carousel-image" ); function showSlide(index) { images.forEach(img => img.style.display = "none" ); images[index].style.display = "block" ; } function prevSlide() { currentIndex = (currentIndex === 0) ? images.length - 1 : currentIndex - 1; showSlide(currentIndex); } function nextSlide() { currentIndex = (currentIndex === images.length - 1) ? 0 : currentIndex + 1; showSlide(currentIndex); } |
3. Bootstrap Image Carousel
Bootstrap provides a carousel component that simplifies implementation.
< div id = "carouselExample" class = "carousel slide" data-bs-ride = "carousel" > < div class = "carousel-inner" > < div class = "carousel-item active" > < img src = "image1.jpg" class = "d-block w-100" alt = "..." > </ div > < div class = "carousel-item" > < img src = "image2.jpg" class = "d-block w-100" alt = "..." > </ div > < div class = "carousel-item" > < img src = "image3.jpg" class = "d-block w-100" alt = "..." > </ div > </ div > < button class = "carousel-control-prev" type = "button" data-bs-target = "#carouselExample" data-bs-slide = "prev" > < span class = "carousel-control-prev-icon" aria-hidden = "true" ></ span > </ button > < button class = "carousel-control-next" type = "button" data-bs-target = "#carouselExample" data-bs-slide = "next" > < span class = "carousel-control-next-icon" aria-hidden = "true" ></ span > </ button > </ div > |
4. Conclusion
Image carousels improve website aesthetics and user engagement. Whether using custom JavaScript or Bootstrap, they are essential for showcasing content dynamically.

Recent Comments