JavaScript in HTML: The Basics
JavaScript is a powerful programming language used to add interactivity and dynamic functionality to web pages. It works alongside HTML and CSS to create engaging user experiences.
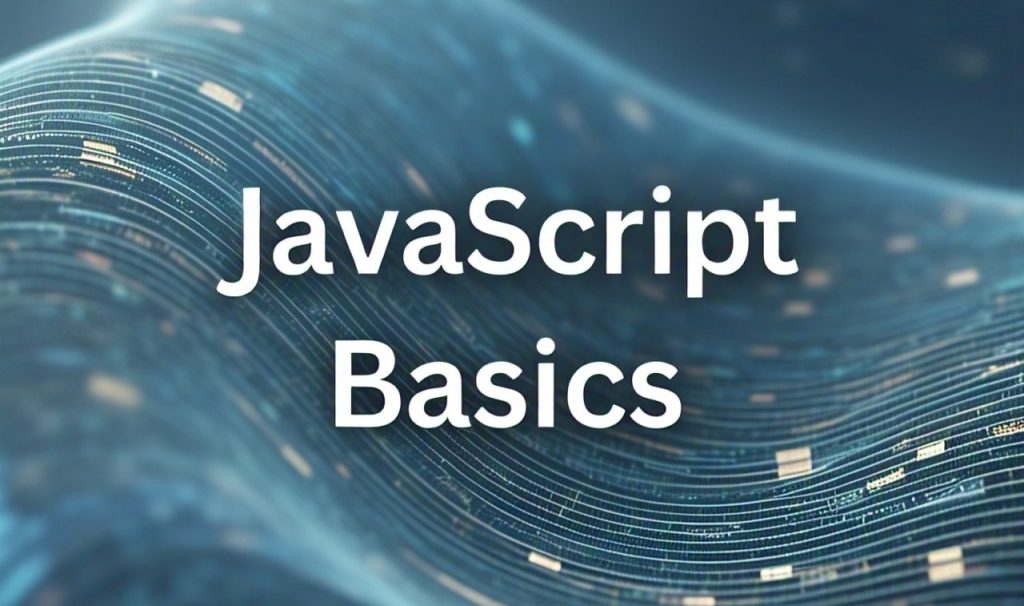
1. What is JavaScript?
JavaScript is a client-side scripting language that allows developers to implement complex features on web pages, such as:
- Interactive forms
- Animations
- Event handling
- Real-time updates
2. How to Include JavaScript in HTML
JavaScript can be included in an HTML document in three ways:
Inline JavaScript
Write JavaScript directly within an HTML element using the onclick
attribute:
<button onclick="alert('Hello, World!')">Click Me</button>
Internal JavaScript
Include JavaScript inside a <script>
tag in the HTML file:
<script>
document.write("Hello, JavaScript!");
</script>
External JavaScript
Create a separate .js
file and link it to the HTML document:
<script src="script.js"></script>
3. JavaScript Syntax Basics
1. Variables
Variables store data values:
var name = "John";
let age = 25;
const PI = 3.14;
2. Data Types
JavaScript supports various data types:
let num = 10; // Number
let text = "Hello"; // String
let isTrue = true; // Boolean
let arr = [1, 2, 3]; // Array
let obj = {name: "John", age: 25}; // Object
4. Functions in JavaScript
Functions are used to perform specific tasks:
function greet(name) {
return "Hello, " + name;
}
console.log(greet("Alice"));
5. JavaScript Events
Events allow interaction with HTML elements:
<button id="myButton">Click Me</button>
<script>
document.getElementById("myButton").addEventListener("click", function() {
alert("Button Clicked!");
});
</script>
6. DOM Manipulation
JavaScript can modify HTML elements dynamically:
document.getElementById("demo").innerHTML = "Hello, JavaScript!";
Example:
<p id="demo">Old Text</p>
<button onclick="changeText()">Change Text</button>
<script>
function changeText() {
document.getElementById("demo").innerHTML = "New Text!";
}
</script>
7. Loops and Conditions
1. Conditional Statements
if (age > 18) {
console.log("Adult");
} else {
console.log("Minor");
}
2. Loops
for (let i = 0; i < 5; i++) {
console.log("Iteration: " + i);
}
8. Conclusion
JavaScript is essential for making web pages interactive. By understanding variables, functions, events, and DOM manipulation, you can create dynamic websites. In the next guide, we will explore Image Carousel in HTML, showcasing how to create sliding image galleries!

Kushagra Kumar Mishra

Latest posts by Kushagra Kumar Mishra (see all)
- Advanced WordPress Topics: Security, Performance, and Scalability (Part-2) - March 10, 2025
- Troubleshooting and Advanced Topics Part-1 - March 10, 2025
- Website Design and Development - March 10, 2025
Recent Comments