Pagination in PHP
Pagination is a technique used to divide large datasets into smaller, manageable chunks and display them across multiple pages. It is commonly used in web applications to improve the user experience and optimize performance by avoiding loading all data at once.
In this blog, we’ll learn how to implement pagination in PHP step by step, including theory, code examples, and best practices.
you may also like
CRUD operations
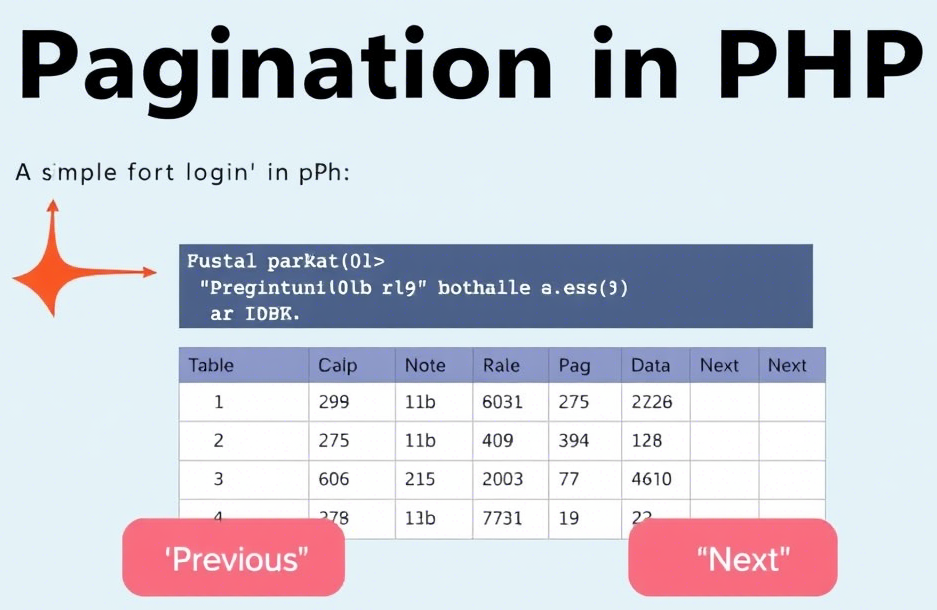
Why Use Pagination?
- Performance: Reduces server load by limiting the number of records displayed per page.
- Usability: Enhances user experience by making data easier to navigate.
- Scalability: Helps manage large datasets effectively.
Prerequisites
- PHP Environment: Ensure PHP is set up using XAMPP or any other local server.
- Database: Use MySQL or another database with a table containing a large dataset.
- Basic Knowledge: Familiarity with PHP, MySQL, and HTML.
Step 1: Create a Sample Database Table
SQL Query to Create Table and Insert Data:
CREATE TABLE products (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
price DECIMAL(10, 2)
);
INSERT INTO products (name, price) VALUES
('Product 1', 100.00),
('Product 2', 200.00),
('Product 3', 150.00),
('Product 4', 120.00),
('Product 5', 250.00),
('Product 6', 300.00),
('Product 7', 400.00),
('Product 8', 500.00);
Step 2: Connect to the Database
Database Configuration File (db.php
):
<?php
$servername = "localhost";
$username = "root";
$password = "";
$database = "testdb";
$conn = new mysqli($servername, $username, $password, $database);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
?>
Step 3: Pagination Logic
Pagination involves calculating the total number of pages and fetching a limited number of records for the current page.
Pagination Variables
- Current Page: Tracks the page the user is on.
- Records per Page: Limits the number of records displayed on each page.
- Offset: Determines where to start fetching records from the database.
Step 4: Fetch and Display Data with Pagination
PHP Code for Pagination (pagination.php
):
<?php
include 'db.php';
// Set the number of records per page
$records_per_page = 3;
// Get the current page from the URL, default to 1
$current_page = isset($_GET['page']) ? (int)$_GET['page'] : 1;
// Calculate the offset
$offset = ($current_page - 1) * $records_per_page;
// Fetch total records
$total_records_query = "SELECT COUNT(*) AS total FROM products";
$total_records_result = $conn->query($total_records_query);
$total_records = $total_records_result->fetch_assoc()['total'];
// Calculate total pages
$total_pages = ceil($total_records / $records_per_page);
// Fetch records for the current page
$query = "SELECT * FROM products LIMIT $offset, $records_per_page";
$result = $conn->query($query);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PHP Pagination</title>
</head>
<body>
<h1>Product List</h1>
<table border="1">
<tr>
<th>ID</th>
<th>Name</th>
<th>Price</th>
</tr>
<?php while ($row = $result->fetch_assoc()) : ?>
<tr>
<td><?php echo $row['id']; ?></td>
<td><?php echo $row['name']; ?></td>
<td><?php echo $row['price']; ?></td>
</tr>
<?php endwhile; ?>
</table>
<div>
<?php for ($i = 1; $i <= $total_pages; $i++) : ?>
<a href="?page=<?php echo $i; ?>">Page <?php echo $i; ?></a>
<?php endfor; ?>
</div>
</body>
</html>
Explanation
- Calculate Total Records: Use
COUNT(*)
to determine the total number of rows in the table. - Set Offset and Limit: Calculate the starting point (
offset
) and limit the number of rows fetched per page. - Generate Links: Dynamically create page links using a loop based on the total number of pages.
- Display Data: Fetch and display the records for the current page.
Best Practices
- Sanitize Input: Use prepared statements to prevent SQL injection when handling user input (e.g.,
$_GET['page']
). - Error Handling: Add error handling for database queries and connections.
- Styling: Use CSS for better presentation of pagination links.
- Dynamic Records per Page: Allow users to select the number of records displayed per page.
Conclusion
Pagination is essential for managing large datasets in web applications. This guide covered the basics of implementing pagination in PHP using MySQL, along with best practices to enhance functionality and security.

Kushagra Kumar Mishra

Latest posts by Kushagra Kumar Mishra (see all)
- Advanced WordPress Topics: Security, Performance, and Scalability (Part-2) - March 10, 2025
- Troubleshooting and Advanced Topics Part-1 - March 10, 2025
- Website Design and Development - March 10, 2025
Recent Comments